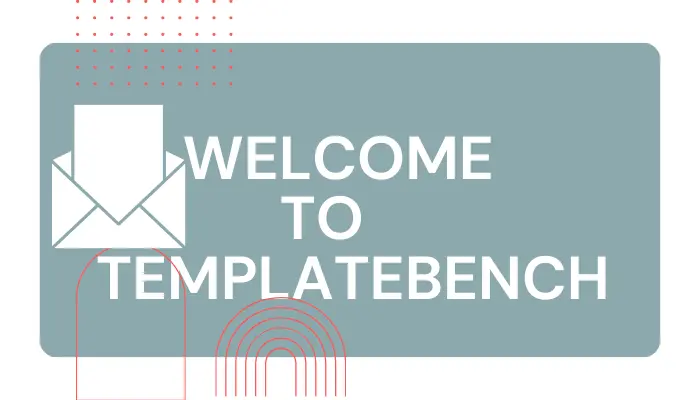
Sending A Warm Welcome: Laravel User Registration And Welcome Email Example
In today's competitive digital landscape, user experience and engagement are paramount. One way to enhance the user experience and engage your users right from the start is by sending a warm welcome email after they register on your website. Laravel, a popular PHP framework, makes this task straightforward with its built-in email functionality and the Laravel Notification system.
In this tutorial, we'll walk you through the process of setting up user registration in a Laravel application and sending a personalized welcome email to new users.
Prerequisites
Before we get started, make sure you have the following prerequisites in place:
- A Laravel application up and running.
- A properly configured mail driver in your Laravel environment.
- Basic familiarity with Laravel's Eloquent ORM and routing.
If you haven't set up a Laravel application yet, you can follow the official Laravel Installation Guide to get started.
Step 1: Configuring Your Mail Settings
The first step is to ensure that your Laravel application is configured to send emails. Open your .env
file and set up the mail configuration according to your email service provider. For this example, we'll use SMTP with Gmail:
MAIL_DRIVER=smtp
MAIL_HOST=smtp.gmail.com
MAIL_PORT=587
MAIL_USERNAME=your_email@gmail.com
MAIL_PASSWORD=your_email_password
MAIL_ENCRYPTION=tls
Replace your_email@gmail.com
and your_email_password
with your actual Gmail credentials. Ensure that you've allowed "Less secure apps" in your Gmail settings if you're using a Gmail account for testing.
Step 2: Creating a Welcome Email Notification
In Laravel, you can send emails using the Notification system. To create a welcome email notification, run the following Artisan command:
php artisan make:notification WelcomeNotification
This command generates a new notification class in the app/Notifications
directory.
Open the WelcomeNotification.php
file and customize the toMail
method to define the content of your welcome email:
use Illuminate\Notifications\Notification;
use Illuminate\Notifications\Messages\MailMessage;
class WelcomeNotification extends Notification
{
public function toMail($notifiable)
{
return (new MailMessage)
->line('Welcome to our website!')
->action('Start Exploring', url('/'))
->line('Thank you for joining us.');
}
}
Feel free to modify the email content, including adding more lines, actions, and buttons to personalize the welcome message.
Step 3: Sending the Welcome Email
Now, let's send the welcome email when a user registers. In your registration controller (typically found in RegisterController.php
), add the following code within the create
method after a successful registration:
use App\Notifications\WelcomeNotification;
protected function create(array $data)
{
$user = User::create([
'name' => $data['name'],
'email' => $data['email'],
'password' => Hash::make($data['password']),
]);
// Send the welcome email notification
$user->notify(new WelcomeNotification());
return $user;
}
With this code in place, when a user successfully registers, the WelcomeNotification
will be sent to their email address, providing a warm welcome and inviting them to explore your website.
Step 4: Testing
It's essential to thoroughly test your registration and welcome email functionality to ensure everything is working as expected. Register a test user on your website, and you should receive a welcome email with the specified content.
Conclusion
Sending a welcome email to users after registration is an excellent way to enhance the user experience, make users feel valued, and introduce them to your platform's features. Laravel simplifies this process with its built-in email capabilities and the Notification system, allowing you to create and send personalized welcome emails effortlessly.
Now that you've learned how to send a warm welcome email to your users, you can further customize and expand this functionality to match your branding and engage your audience effectively.
In future articles, we'll explore more advanced features of Laravel's email and notification system to help you create a seamless and engaging user onboarding experience. Stay tuned!
Thank you for joining us on this journey to improve user engagement and experience in your Laravel applications. Happy coding!