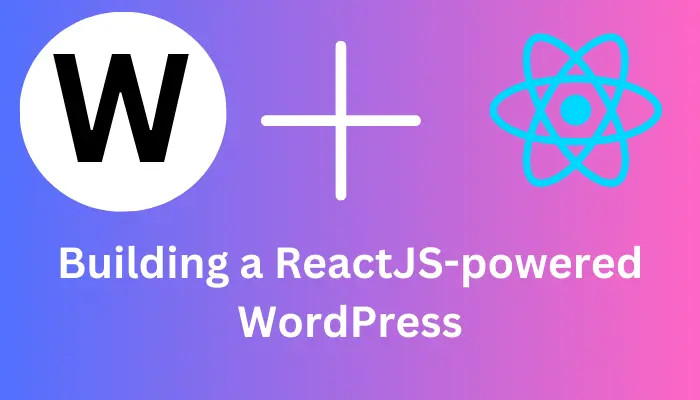
Building A ReactJS-powered WordPress Plugin: A Step-by-Step Guide
Introduction:
Welcome to the exciting realm of integrating ReactJS into a WordPress plugin! In this step-by-step guide, we'll walk through the creation of a dynamic WordPress plugin that leverages the power of ReactJS. Whether you're a developer eager to enhance your WordPress site or someone venturing into the world of web development, this guide is designed to make the process accessible and insightful.
**1. Prerequisites:
Before we start, ensure you have the following tools installed:
- Node.js
- npm (Node Package Manager)
- WordPress installation
**2. Setting Up the Development Environment:
Open your terminal and navigate to your WordPress plugins directory. Create a new directory for your plugin:
cd wp-content/plugins
mkdir my-react-plugin
cd my-react-plugin
**3. Initializing React App:
Inside your plugin directory, initialize a new React app:
npx create-react-app my-react-app
cd my-react-app
This creates a new React app within your WordPress plugin directory.
**4. Connecting to WordPress:
Open the src/App.js
file in your React app and replace its content with a basic component:
import React, { useState, useEffect } from 'react';
function App() {
const [data, setData] = useState([]);
useEffect(() => {
// Fetch data from your WordPress site using the REST API
fetch('https://yourwordpresssite.com/wp-json/wp/v2/posts')
.then((response) => response.json())
.then((result) => setData(result))
.catch((error) => console.error('Error fetching data:', error));
}, []);
return (
<div>
<h1>WordPress React Plugin</h1>
<ul>
{data.map((post) => (
<li key={post.id}>{post.title.rendered}</li>
))}
</ul>
</div>
);
}
export default App;
This simple component fetches and displays a list of posts from your WordPress site using the REST API.
**5. Enqueuing React App in WordPress:
Now, let's enqueue our React app in the WordPress plugin. Create a new PHP file in your plugin directory (e.g., my-react-plugin.php
) with the following content:
<?php
/*
Plugin Name: My React Plugin
*/
function enqueue_react_app() {
wp_enqueue_script('my-react-app', plugins_url('/my-react-app/build/static/js/main.js', __FILE__), array(), '1.0', true);
}
add_action('wp_enqueue_scripts', 'enqueue_react_app');
This code enqueues the React app script in your WordPress plugin.
**6. Activate Your Plugin:
Navigate to your WordPress admin dashboard, go to Plugins, and activate "My React Plugin."
**7. See it in Action:
Create a new page in WordPress and add the following shortcode:
[my_react_plugin]
Visit the page, and you should see your React-powered WordPress plugin in action, displaying a list of posts from your site.
**8. Customizing and Expanding:
Now that you have a basic setup, feel free to customize and expand your React app. Add more components, fetch additional data, and integrate interactive features to create a truly dynamic WordPress plugin.
Conclusion:
Congratulations! You've successfully built a ReactJS-powered WordPress plugin. This step-by-step guide provides a foundation for further exploration and customization. Use this newfound knowledge to enhance your WordPress site with dynamic and engaging React-powered features.
Happy coding!